How to reduce Selenium Test Execution time by disabling images in Chrome, Edge and Firefox.
In this blog I will be sharing how to disable images in browsers like Google Chrome, Edge and Firefox to reduce the Selenium test execution time. I will be sharing two methods to disable images in Chrome and Edge and a single method to disable images in Firefox.
disableImagesInChromeMethodOne()
public static void disableImagesInChromeMethodOne() {
chromeOptions = new ChromeOptions();
chromeOptions.addArguments("--blink-settings=imagesEnabled=false");
driver = new ChromeDriver(chromeOptions);
driver.manage().window().maximize();
driver.get("https://www.amazon.in/");
}
disableImagesInChromeMethodTwo()
public static void disableImagesInChromeMethodTwo() {
chromeOptions = new ChromeOptions();
Map<String, Object> prefs = new HashMap<String, Object>();
prefs.put("profile.managed_default_content_settings.images", 2);
chromeOptions.setExperimentalOption("prefs", prefs);
driver = new ChromeDriver(chromeOptions);
driver.manage().window().maximize();
driver.get("https://www.amazon.in/");
}
disableImagesInEdgeMethodOne()
public static void disableImagesInEdgeMethodOne() {
edgeOptions = new EdgeOptions();
edgeOptions.addArguments("--blink-settings=imagesEnabled=false");
driver = new EdgeDriver(edgeOptions);
driver.manage().window().maximize();
driver.get("https://www.amazon.in/");
}
disableImagesInEdgeMethodTwo()
public static void disableImagesInEdgeMethodTwo() {
edgeOptions = new EdgeOptions();
Map<String, Object> prefs = new HashMap<String, Object>();
prefs.put("profile.managed_default_content_settings.images", 2);
edgeOptions.setExperimentalOption("prefs", prefs);
driver = new EdgeDriver(edgeOptions);
driver.manage().window().maximize();
driver.get("https://www.amazon.in/");
}
disableImagesInFirefox()
public static void disableImagesInFirefox() {
firefoxOptions = new FirefoxOptions();
firefoxOptions.addPreference("permissions.default.image", 2);
driver = new FirefoxDriver(firefoxOptions);
driver.manage().window().maximize();
driver.get("https://www.amazon.in/");
}
DisableImagesInBrowsers — complete java class code
package com.tests;
import java.util.HashMap;
import java.util.Map;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.chrome.ChromeOptions;
import org.openqa.selenium.edge.EdgeDriver;
import org.openqa.selenium.edge.EdgeOptions;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.openqa.selenium.firefox.FirefoxOptions;
public class DisableImagesInBrowsers {
private static WebDriver driver;
private static ChromeOptions chromeOptions;
private static EdgeOptions edgeOptions;
private static FirefoxOptions firefoxOptions;
public static void main(String[] args) throws InterruptedException {
disableImagesInChromeMethodOne();
disableImagesInChromeMethodTwo();
disableImagesInEdgeMethodOne();
disableImagesInEdgeMethodTwo();
disableImagesInFirefox();
}
public static void disableImagesInChromeMethodOne() {
chromeOptions = new ChromeOptions();
chromeOptions.addArguments("--blink-settings=imagesEnabled=false");
driver = new ChromeDriver(chromeOptions);
driver.manage().window().maximize();
driver.get("https://www.amazon.in/");
}
public static void disableImagesInChromeMethodTwo() {
chromeOptions = new ChromeOptions();
Map<String, Object> prefs = new HashMap<String, Object>();
prefs.put("profile.managed_default_content_settings.images", 2);
chromeOptions.setExperimentalOption("prefs", prefs);
driver = new ChromeDriver(chromeOptions);
driver.manage().window().maximize();
driver.get("https://www.amazon.in/");
}
public static void disableImagesInEdgeMethodOne() {
edgeOptions = new EdgeOptions();
edgeOptions.addArguments("--blink-settings=imagesEnabled=false");
driver = new EdgeDriver(edgeOptions);
driver.manage().window().maximize();
driver.get("https://www.amazon.in/");
}
public static void disableImagesInEdgeMethodTwo() {
edgeOptions = new EdgeOptions();
Map<String, Object> prefs = new HashMap<String, Object>();
prefs.put("profile.managed_default_content_settings.images", 2);
edgeOptions.setExperimentalOption("prefs", prefs);
driver = new EdgeDriver(edgeOptions);
driver.manage().window().maximize();
driver.get("https://www.amazon.in/");
}
public static void disableImagesInFirefox() {
firefoxOptions = new FirefoxOptions();
firefoxOptions.addPreference("permissions.default.image", 2);
driver = new FirefoxDriver(firefoxOptions);
driver.manage().window().maximize();
driver.get("https://www.amazon.in/");
}
}
After executing the above java class 2 browser windows of Chrome, Edge and 1 browser window of Firefox will be opened respectively with Amazon website having images disabled.
Screenshots of browsers after disabling images:


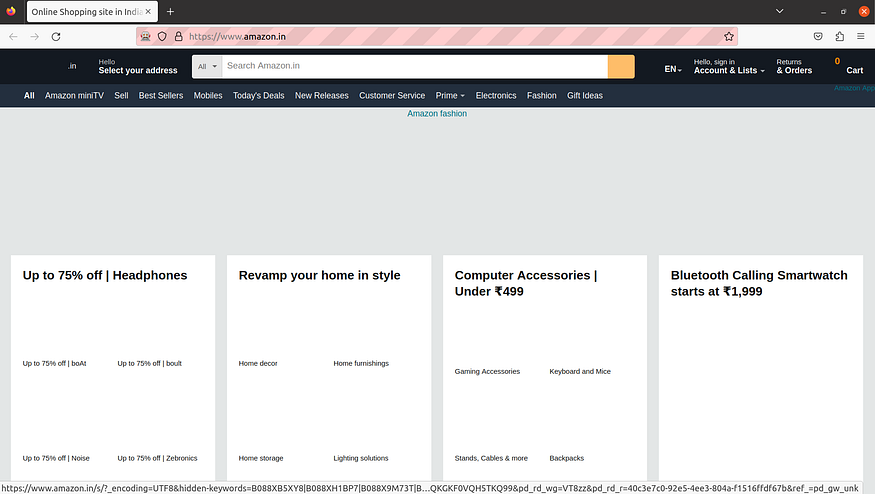
Note: I have used Selenium Version 4.12.1, Google Chrome Version 117.0.5938.88, Microsoft Edge Version 117.0.2045.31 and Mozilla Firefox Version 117.0.1
Comments
Post a Comment